Python’s functions are the language’s underlying structure. They allow for bigger programs to be broken down into smaller, more manageable pieces. They also assist with code reusability, which means that a block of code can be used multiple times without having to rewrite it each time.
This is another benefit of using modules in Datacamp. Because Python functions can be called at any place in the program and can also return values, they are extremely versatile tools that can be used to solve a wide variety of complex programming problems.
Defining a Python Function
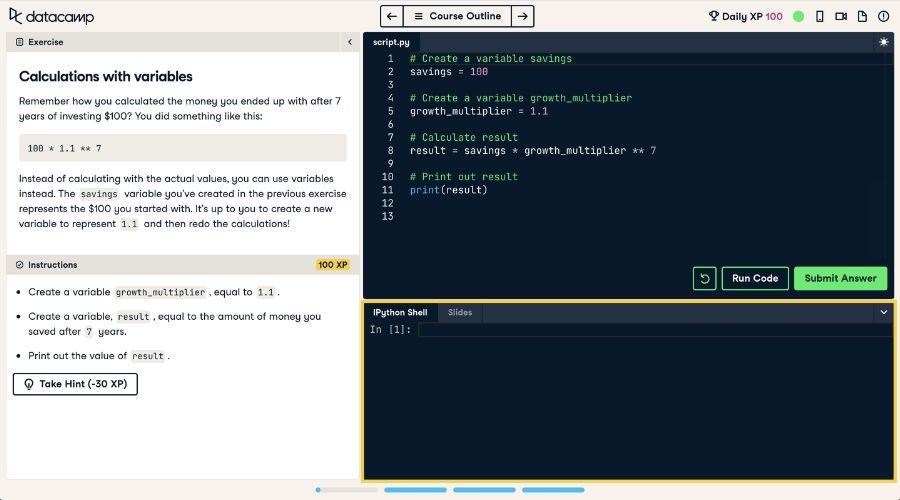
Defining a Python Function | neonpolice
A function can be defined in Python by beginning with the “def” keyword, then following it with the function’s name, and ending with a pair of parentheses. It is possible for the parentheses to contain parameters that are not required for the function to carry out its work. The body of the function is indented, and it may contain any number of statements—including conditional statements, loops, and other functions—as well as any other kind of statement.
The following is an illustration of a straightforward Python procedure that outputs a message:
def print_message():
print(“Hello, world!”)
In this example, we defined a function called “print_message” that doesn’t take any arguments and simply prints the message “Hello, world!” when called.
Function Parameters and Arguments
Python functions have the ability to take one or more parameters, which are essentially data stubs that stand in for information that the function requires in order to carry out its purpose. The values that are passed to the parameters of a function are referred to as its arguments when the function is invoked. Both the parameters and the arguments can be of any data format, such as strings, integers, floats, or even something completely different.
The following is an illustration of a Python function that receives two parameters and outputs the sum of those parameters:
def add_numbers(x, y):
return x + y
The “add_numbers” method shown here accepts the values “x” and “y” as its input arguments. The function body returns the sum of these two parameters.
Calling a Python Function
To call a Python function, we simply use the function name followed by parentheses. If the function takes arguments, we pass them inside the parentheses.
Here’s an example of calling the “print_message” function we defined earlier:
print_message()
When we call this function, it will print the message “Hello, world!” to the console.
Here’s an example of calling the “add_numbers” function with arguments:
result = add_numbers(3, 5)
print(result)
In this example, we called the “add_numbers” function with the arguments 3 and 5. The function returned their sum, which we stored in a variable called “result”. Our next step was to output the number of “results” to the console.
Returning Values from a Python Function
Python functions can also return values using the “return” keyword. When a function returns a value, it can be assigned to a variable or used in any other way that a value of that data type can be used.
Here’s an example of a Python function that returns the square of a number:
def square(x):
return x **
In this example, we defined a function called “square” that takes one parameter, “x”. The function body returns the square of “x”.
We can call this function and assign its return value to a variable like this:
result = square(4)
print(result)
In this example, we called the “square” function with the argument 4. The function returned the square of 4 (which is 16), which we stored in a variable called “result”. Following that, we output to the console the number that was stored in the “result” variable.
What is the built-in Python function?
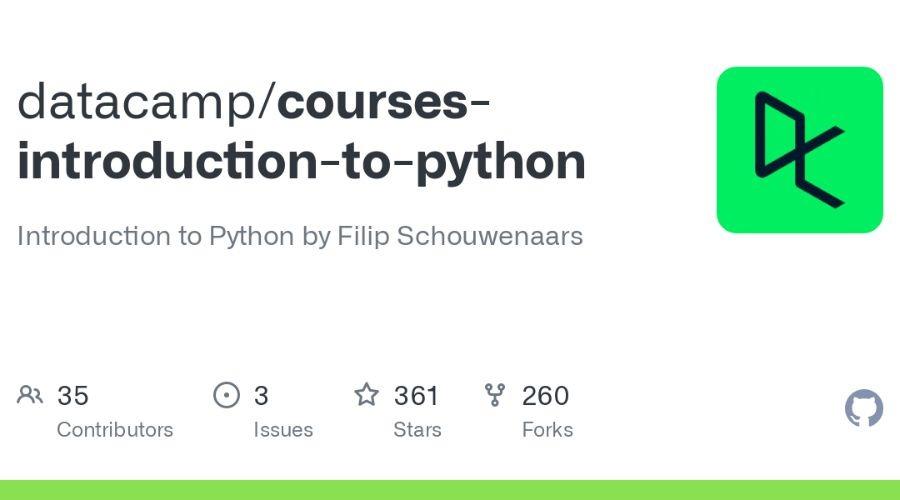
What is the built-in Python function | neonpolice
Python is a high-level programming language that can be interpreted and comes with a large number of built-in functions. These functions have already been declared and defined, so a Python application does not need to make any additional declarations or modifications before using them. Programming is made more user-friendly, effective, and enjoyable thanks to Python’s built-in functions, which are an important component of the Python programming language.
Definition
Built-in functions in Python are those functions that are included in the standard package that comes with the programming language. These functions have already been specified and can be used in a program without the need for any additional import statements or library installations because they are predefined functions.
The purpose of a program’s built-in functions is to provide frequently used functionalities that are necessary for the majority of programs. The functionality of these functions has been fine-tuned, and they have been implemented entirely in C or Python, which makes them incredibly quick and effective.
Why are Built-in Functions Important?
In Python programming, built-in functions are very essential for a number of different reasons. To begin, they reduce the amount of time and effort required to accomplish a task by offering pre-defined functionality. This functionality can be used immediately within a program without the need for any previous declaration or definition.
The second advantage of using built-in functions is that, because they have already been optimized for performance, they can be called much more quickly than user-defined functions. This is because C or Python itself is used to construct built-in functions, which results in the functions having a higher level of efficiency.
Last but not least, built-in functions offer a consistent method of completing frequently performed duties, which makes the code more readable and straightforward to comprehend. This is due to the fact that built-in functions have standardized titles and syntax, which makes it simpler to identify them and put them to use.
Conclusion
In a nutshell, Python functions provide programmers with a wide variety of advantages. Large programs can be broken down into smaller, more manageable portions using them, which makes it much simpler to maintain and update the code. In addition to this, functions make it possible to reuse code, which, over the course of a project, can save a substantial amount of time and effort. For more information, visit Neonpolice.